基于Python实现RLE格式分割标注文件的格式转换-深度学习数据处理
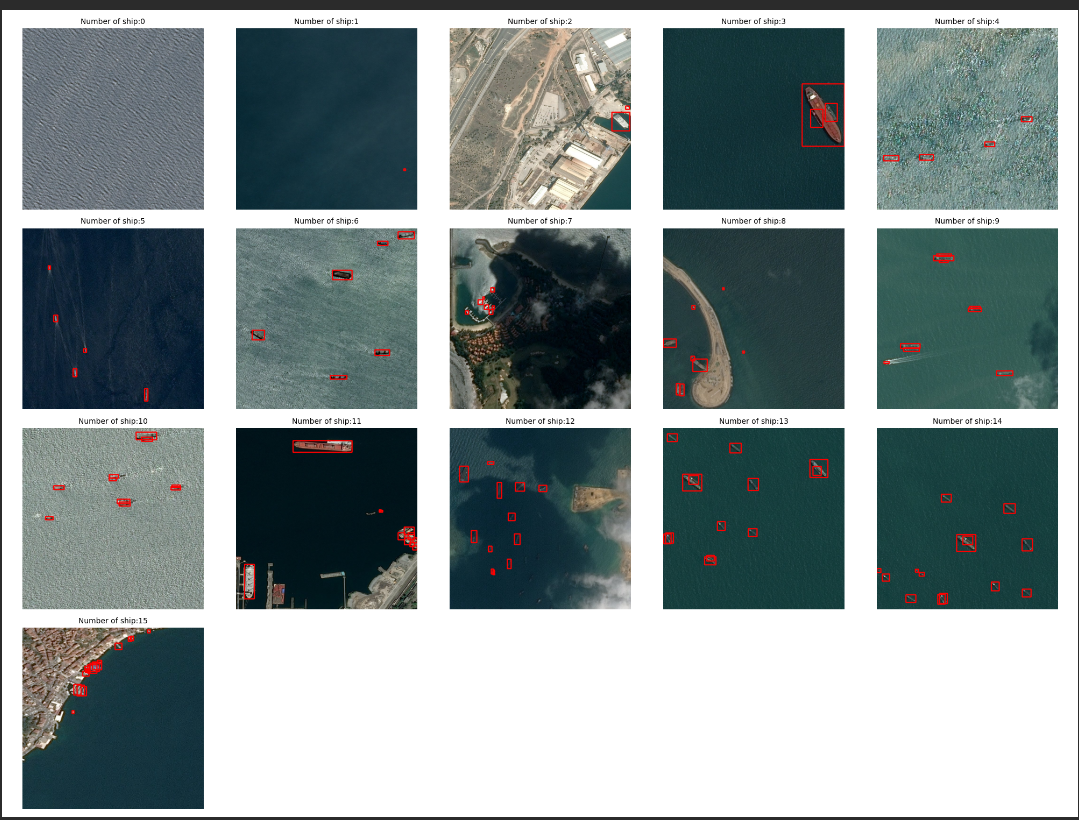
基于Python实现RLE格式分割标注文件的格式转换-深度学习数据处理
ytkzRLE (Run-Length Encoding) 是一种简单的无损数据压缩算法,常用于压缩图像数据。在图像处理中,特别是位图图像的存储和传输方面,RLE 可以有效地减少存储空间或带宽需求。
RLE 的基本思想是将连续出现的相同数据用一个计数和该值来代替。例如,在黑白图像中,如果有一系列连续的白色像素(假设用数字 1 表示),那么这一系列的像素可以用一个计数和数字 1 来表示。
RLE 编码示例
rle = "1 2 4 3 7 1 9 5"
shape = (10, 10) # 图像尺寸为 10x10
这里的 RLE 编码 "1 2 4 3 7 1 9 5"
表示如下信息:
- 从位置 1 开始有 2 个连续的像素;
- 从位置 4 开始有 3 个连续的像素;
- 从位置 7 开始有 1 个连续的像素;
- 从位置 9 开始有 5 个连续的像素。
模拟实战
import numpy as np
import matplotlib.pyplot as plt
def rle_decode(rle, shape, colors):
"""
解码 RLE 编码到彩色图像。
:param rle: RLE 编码字符串
:param shape: 图像尺寸 (height, width)
:param colors: 颜色列表,对应于 RLE 中的每个段落
:return: 彩色图像
"""
s = rle.split()
starts, lengths = [np.asarray(x, dtype=int) for x in (s[0:][::2], s[1:][::2])]
starts -= 1 # RLE 通常以 1 为索引,这里转换为从 0 开始
ends = starts + lengths
img = np.zeros(shape + (3,), dtype=np.uint8) # 3 通道 RGB 图像
for idx, (lo, hi) in enumerate(zip(starts, ends)):
if hi > shape[0] * shape[1]:
hi = shape[0] * shape[1]
img[np.unravel_index(range(lo, hi), shape)] = colors[idx]
return img
# 给定的 RLE 编码
rle = "1 2 4 3 7 1 9 5"
shape = (10, 10) # 图像尺寸为 10x10
# 定义颜色
colors = [(255, 0, 0), (0, 255, 0), (0, 0, 255), (255, 255, 0)]
# 解码 RLE 编码
img = rle_decode(rle, shape, colors)
# 可视化
plt.imshow(img)
plt.title('Decoded Color Image from RLE')
plt.axis('off')
plt.show()
airbus-ship实战
def rle2bbox(rle, shape):
# 假设RLE字符串中的元素由空格分隔
a = np.fromiter(rle.split(), dtype=np.uint32) # 根据实际情况选择合适的整数类型
a = a.reshape((-1, 2)) # 转换为(start, length)对
a[:, 0] -= 1 # 将1-indexed转换为0-indexed
# 计算y坐标
y0 = a[:, 0] % shape[0]
y1 = np.minimum(y0 + a[:, 1], shape[0]) # 防止y1超出图像高度
y0 = np.min(y0)
y1 = np.max(y1)
# 计算x坐标
x0 = a[:, 0] // shape[0]
x1 = np.minimum((a[:, 0] + a[:, 1] - 1) // shape[0], shape[1] - 1) # 防止x1超出图像宽度
x0 = np.min(x0)
x1 = np.max(x1)
# 计算边界框的中心和尺寸(不归一化)
xC = (x0 + x1) / 2
yC = (y0 + y1) / 2
h = y1 - y0 + 1
w = x1 - x0 + 1
# 如果需要归一化,可以使用以下方式:
xC /= shape[1]
yC /= shape[0]
h /= shape[0]
w /= shape[1]
return [xC, yC, h, w]