GitHub 项目 OpenFreeMap
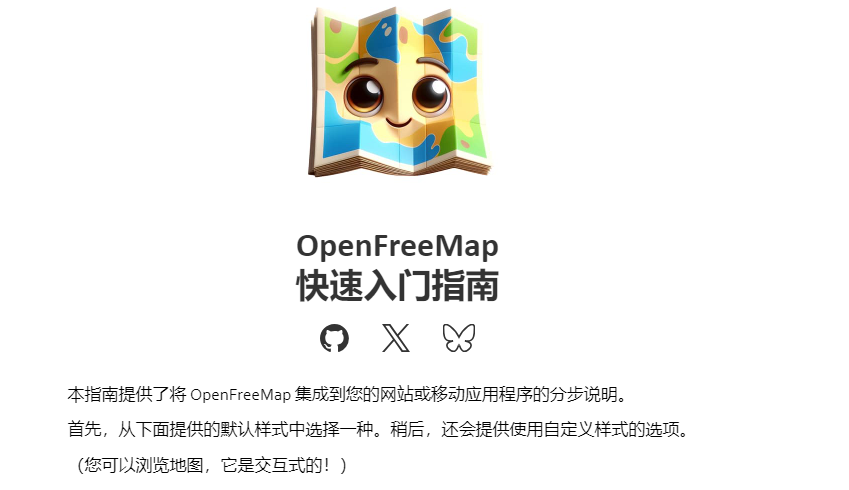
GitHub 项目 OpenFreeMap
ytkz代码
import sys
import os
import subprocess
import threading
import shutil
from PyQt5.QtWidgets import QApplication, QMainWindow, QWidget, QVBoxLayout, QHBoxLayout, QSpinBox
from PyQt5.QtGui import QIcon
from PyQt5.QtCore import Qt
from qfluentwidgets import (LineEdit, ComboBox, PushButton, TextEdit, BodyLabel,
MessageBox, CheckBox, IndeterminateProgressBar)
class YTDLPInterface(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("yt-dlp 超清下载器")
self.resize(800, 600)
self.setWindowIcon(QIcon("icon.png"))
container = QWidget()
self.setCentralWidget(container)
layout = QVBoxLayout(container)
layout.setContentsMargins(25, 25, 25, 25)
layout.setSpacing(20)
self.progress_bar = IndeterminateProgressBar()
self.progress_bar.hide()
layout.addWidget(self.progress_bar)
self.url_entry = LineEdit()
self.url_entry.setPlaceholderText("请输入视频或播放列表URL(支持多个URL,用空格分隔)")
self.url_entry.setClearButtonEnabled(True)
self.url_entry.setText('https://www.bilibili.com/video/BV1iW9RYWEiJ/')
layout.addWidget(BodyLabel("视频/播放列表URL:"))
layout.addWidget(self.url_entry)
self.format_combo = ComboBox()
self.format_combo.addItems([
"最高质量(自动合并音视频)",
"仅最佳视频",
"仅最佳音频"
])
layout.addWidget(BodyLabel("下载格式:"))
layout.addWidget(self.format_combo)
self.path_entry = LineEdit()
self.path_entry.setPlaceholderText("默认路径:./downloads")
self.path_entry.setClearButtonEnabled(True)
layout.addWidget(BodyLabel("保存路径:"))
layout.addWidget(self.path_entry)
self.subtitle_checkbox = CheckBox("下载字幕(自动选择英简中文字幕)")
self.subtitle_checkbox.setChecked(True)
layout.addWidget(self.subtitle_checkbox)
self.metadata_checkbox = CheckBox("添加元数据信息(需要ffmpeg)")
self.metadata_checkbox.setChecked(True)
layout.addWidget(self.metadata_checkbox)
playlist_layout = QHBoxLayout()
self.playlist_checkbox = CheckBox("下载整个播放列表")
self.playlist_checkbox.setChecked(False)
playlist_layout.addWidget(self.playlist_checkbox)
self.playlist_limit_label = BodyLabel("下载数量限制:")
self.playlist_limit = QSpinBox()
self.playlist_limit.setRange(1, 1000)
self.playlist_limit.setValue(100)
self.playlist_limit.setEnabled(False)
playlist_layout.addWidget(self.playlist_limit_label)
playlist_layout.addWidget(self.playlist_limit)
playlist_layout.addStretch()
layout.addLayout(playlist_layout)
self.playlist_checkbox.stateChanged.connect(self.toggle_playlist_limit)
button_layout = QHBoxLayout()
self.list_formats_button = PushButton("列出可用格式")
self.list_formats_button.clicked.connect(self.list_formats)
button_layout.addWidget(self.list_formats_button)
self.download_button = PushButton("开始下载")
self.download_button.clicked.connect(self.start_download)
button_layout.addWidget(self.download_button)
layout.addLayout(button_layout)
layout.addWidget(BodyLabel("下载日志:"))
self.output_text = TextEdit()
self.output_text.setReadOnly(True)
layout.addWidget(self.output_text)
def toggle_playlist_limit(self, state):
self.playlist_limit.setEnabled(state == Qt.Checked)
def build_command(self, url, list_formats=False):
command = ["yt-dlp", "--newline"]
if list_formats:
command.append("--list-formats")
else:
format_choice = self.format_combo.currentText()
if format_choice == "最高质量(自动合并音视频)":
# Use a fallback format to handle unavailable streams
if 'bilibili' in url:
command += [
"-f", "bestvideo+bestaudio/best", # Fallback to 'best' if bv+ba fails
"--merge-output-format", "mp4",
"--embed-thumbnail",
"--embed-metadata"
]
else:
command += [
"-f", "bv[ext=webm]+ba[ext=m4a]",
"--merge-output-format", "mp4",
"--embed-thumbnail",
"--embed-metadata"
]
if self.subtitle_checkbox.isChecked():
command.extend(["--write-auto-subs", "--sub-langs", "en,zh-Hans"])
if self.metadata_checkbox.isChecked():
command += ["--embed-metadata", "--embed-thumbnail"]
if self.playlist_checkbox.isChecked():
command.append("--yes-playlist")
max_videos = self.playlist_limit.value()
command.extend(["--playlist-items", f"1-{max_videos}"])
else:
command.append("--no-playlist")
output_path = self.path_entry.text() or "./downloads"
os.makedirs(output_path, exist_ok=True)
command += ["-o", f"{output_path}/%(playlist_title)s/%(title)s [%(id)s].%(ext)s"]
command.append(url)
return command
def start_download(self):
urls = self.url_entry.text().split()
if not urls:
MessageBox("错误", "请输入至少一个视频或播放列表URL", self).exec()
return
if not self.check_dependencies():
return
self.download_button.setEnabled(False)
self.list_formats_button.setEnabled(False)
self.progress_bar.show()
thread = threading.Thread(target=self.run_downloads, args=(urls,))
thread.start()
def list_formats(self):
urls = self.url_entry.text().split()
if not urls:
MessageBox("错误", "请输入至少一个视频或播放列表URL", self).exec()
return
if not shutil.which("yt-dlp"):
MessageBox("依赖缺失", "缺少yt-dlp,请安装后重试", self).exec()
return
self.output_text.clear()
self.download_button.setEnabled(False)
self.list_formats_button.setEnabled(False)
self.progress_bar.show()
thread = threading.Thread(target=self.run_list_formats, args=(urls,))
thread.start()
def check_dependencies(self):
missing = []
if not shutil.which("yt-dlp"):
missing.append("yt-dlp(主程序)")
if self.format_combo.currentText() != "仅最佳音频" and not shutil.which("ffmpeg"):
missing.append("ffmpeg(视频处理)")
if missing:
msg = "缺少必要组件:\n" + "\n".join(missing) + "\n\n请安装后重试"
MessageBox("依赖缺失", msg, self).exec()
return False
return True
def run_list_formats(self, urls):
try:
for url in urls:
command = self.build_command(url.strip(), list_formats=True)
self.output_text.append(f"正在获取格式列表:{url}")
self.output_text.append("执行命令: " + " ".join(command))
with subprocess.Popen(
command,
stdout=subprocess.PIPE,
stderr=subprocess.STDOUT,
bufsize=1,
universal_newlines=True,
encoding='utf-8',
errors='replace'
) as process:
for line in process.stdout:
self.output_text.append(line.strip())
QApplication.processEvents()
process.wait()
if process.returncode == 0:
self.output_text.append(f"✓ 格式列表获取完成:{url}\n")
else:
self.output_text.append(f"✗ 获取格式失败:{url} (错误码: {process.returncode})\n")
except Exception as e:
self.output_text.append(f"严重错误: {str(e)}")
finally:
self.download_button.setEnabled(True)
self.list_formats_button.setEnabled(True)
self.progress_bar.hide()
def run_downloads(self, urls):
try:
for url in urls:
command = self.build_command(url.strip())
self.output_text.append(f"开始下载:{url}")
self.output_text.append("执行命令: " + " ".join(command))
with subprocess.Popen(
command,
stdout=subprocess.PIPE,
stderr=subprocess.STDOUT,
bufsize=1,
universal_newlines=True,
encoding='utf-8',
errors='replace'
) as process:
for line in process.stdout:
self.output_text.append(line.strip())
QApplication.processEvents()
process.wait()
if process.returncode == 0:
self.output_text.append(f"✓ 下载完成:{url}\n")
else:
self.output_text.append(f"✗ 下载失败:{url} (错误码: {process.returncode})\n")
self.output_text.append("提示:尝试使用'列出可用格式'检查支持的格式,或调整下载设置。\n")
except Exception as e:
self.output_text.append(f"严重错误: {str(e)}")
finally:
self.download_button.setEnabled(True)
self.list_formats_button.setEnabled(True)
self.progress_bar.hide()
if __name__ == "__main__":
app = QApplication(sys.argv)
window = YTDLPInterface()
window.show()
sys.exit(app.exec())